Arduino Due support
From the beginning DiveIno was built based on the Arduino Mega 2560 microcontroller board. The main reasons of this decision were the followings:
- 256 KB flash memory to contain a big sketch like DiveIno
- Be able to drive the 3.2 inch TFT IPS 480 x 320 262K Color Full-Angle LCD Module
- Stable and affordable clones are available
However during DiveIno development I faced some inconveniences:
- Slow processing and response lags (e.g. on the IR sensor)
- Slow display refresh rate on the TFT panel
In real life, if you navigate in the main menu or switch into Gauge or Dive modes, you can experience some slowness. In order to overcome these problems I can see two possible solutions:
- Decouple the UI presentation logic calculation from the microcontroller
- Upgrade to a faster microcontroller
The first way is done by ITEAD Studio in their Nextion TFT LCD product range. A powerful ARM 7 CPU integrated onto the display PCB is responsible for all presentation logic calculation. The connected microcontroller board - like Arduino Mega 2560 - only provides the required data through a serial interface.
The second way is to change the board to something more powerful. In the last few years the Arduino boards started to get migrated to 32 bit ARM CPUs. In the Arduino Uno product line first came the Arduino M0 Pro then recently the Arduino PRIMO. The same evolution took place in the Mega product line. First came out the Arduino DUE, which was followed by the Arduino Star Otto.
I ordered a 3.5” Nextion Enhanced model from ITEAD Studio, but it is still under delivery. Once it arrives I will have a look.
In the meantime I decided to do an upgrade onto the Arduino DUE board. I bought one from Malnapc in 2015, so I had it on the shelf. The other reason why I picked this board from the Arduino Mega product line, because it is on the market for a few years. During this time, third party developers ported their libraries to support Due or released new libraries.
My other requirement was to support both Arduino Mega 2560 and Arduino DUE boards on the same codebase.
Libraries
The first step in the migration was to change the board type in the development IDE. I use Sloeber formerly known as Arduino Eclipse Plugin for development, which supports both boards through its Board Manager. The same thing can be done in the official Arduino IDE as well.
If you try to compile the original DiveIno codebase, you will notice quite soon that it won’t compile with Due! The main reason is that some third party libraries, which works with Mega doesn’t work with Due. If you have a compile error, it is a clear indication of this problem. The other one is when your code compiles, but it doesn’t work.
Based on these experiences I decided to put together a test platform for the migration. DiveIno relies on the following components:
- Piezo buzzer to make a beep
- IR receiver to support user interaction
- Pressure sensor
- Lipo battery power supply and state of charge indication
- RTC (Real Time Clock) to provide date and time information
- TFT LCD display
- SD card to store settings and dive data
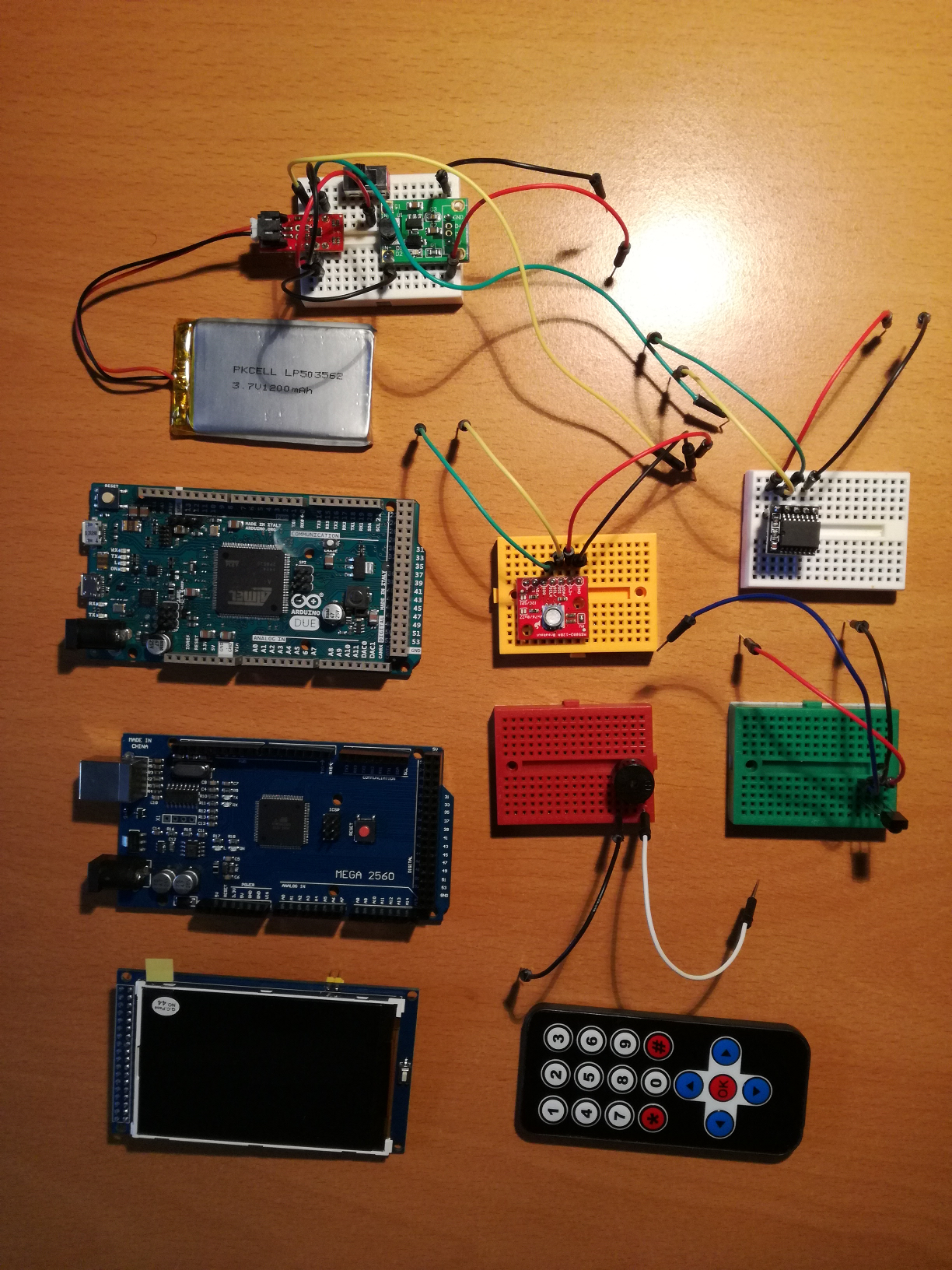
The final goal of the migration was to be able to support these components with suitable libraries for both on Arduino Mega 2560 and Arduino DUE boards.
Audio
The first issue which I faced with, is that the Arduino tone() method is not implemented on Due. I use this to generate a beep sound. I had to find a library which works on Due. Fortunately the Timer Free Tone library supports everything what I need.
The downside of this library is that it blocks Arduino sketch execution until the sound play gets finished. For sure it is not the desired behavior on the slower Mega. It would be good to preserve the orignal parallel execution based on a timer interrupt on Mega and use the new library only on Due.
It can be done with C++ preprocessor conditional compilation statements like this:
1 |
|
As it can be seen above the Timer Free Tone library was only included in case of Due. The following beep() method implements correctly the sound generation on both boards:
1 | void beep() |
IR receiver
Unfortunately the Arduino IRremote library doesn’t support Arduino Due. Thankfully enternoescape 2 years ago forked this library and added Due support. The downside is that this library doesn’t maintained any more, so new updates are unavailable. Anyway it does the job and this is what we are looking for.
The Arduino IRremote Due is a drop in replacement of the original library. Only the include statement has to be changed.
Pressure sensor
Sparkfun provides the MS5803-14BA Arduino Library to the SparkFun Pressure Sensor Breakout. Unfortunately this library is not compatible with Arduino Due. Instead the MS5803_14 library has to be used. It provides the pressure and temperature information similarly how the Sparkfun library does.
Power supply
Arduino Due requires more power (100 mAh) than the Arduino Mega board (38 mAh). If you add the TFT LCD and the sensors, it will imply quite high power supply requirement. The original SparkFun LiPo Fuel Gauge based power supply can’t cope with such a demand. It can power the Arduino Mega board, but fails with the Due. The symptom is that the board becomes unstable.
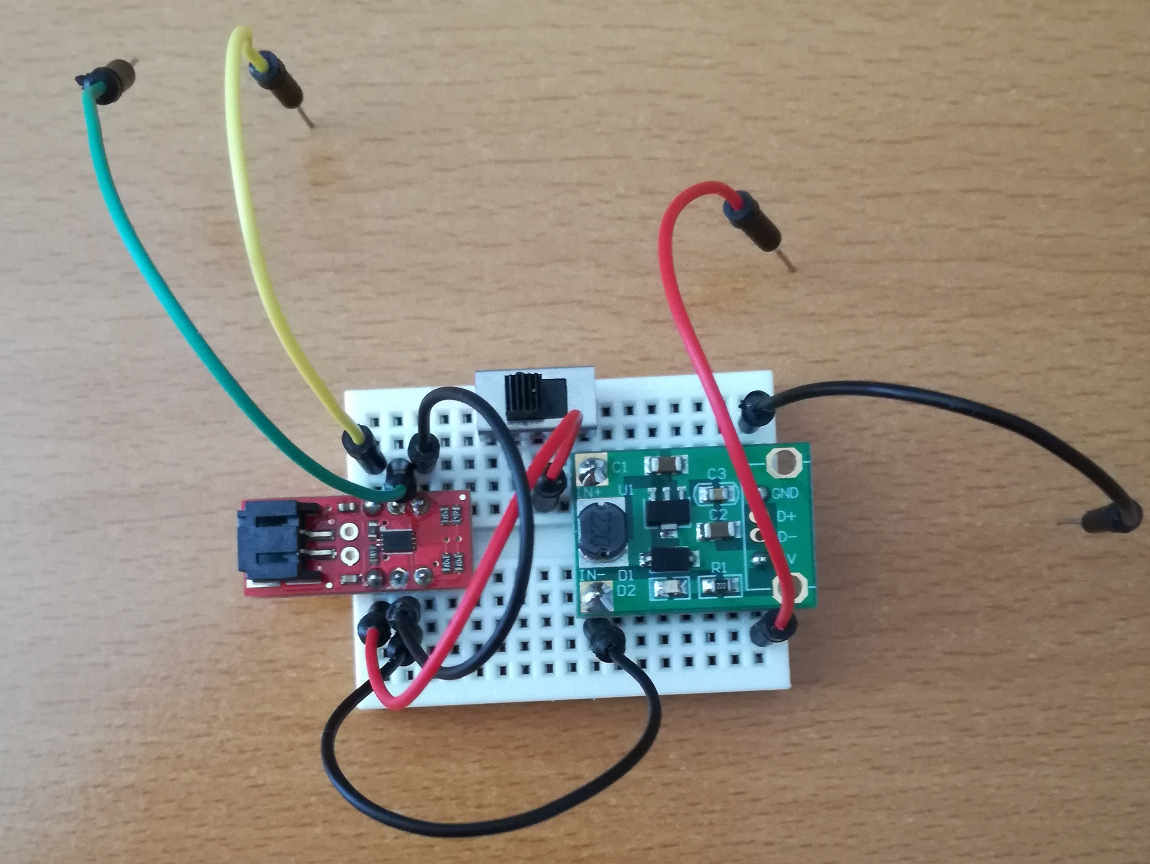
The solution is to use the Adafruit PowerBoost 500 Charger module in conjunction with the LiPo Fuel Gauge. This combination can provide enough power to the Arduino Due based DiveIno setup.
Fortunately the MAX17043 library supports Mega and Due out of the box, so no change in the code is required.
Real Time Clock
The other compile error, which I got at the start is from the DS3232RTC library. Based on a post the library author said the followings:
This library was written for the AVR MCUs and is known not to compile for the ARM architecture. No current plans to address this, sorry.
This is a really straight indication about this library has to get replaced. The candidate is the
DS1307RTC library. Besides the DS1307 chip, it supports the DS3231 real time clock chip as well on both Arduino Mega and Due.
I had to spend quite some time to make this replacement work. The solution is based on a similar technique, which I used in case of audio support. The details can be found in the RTC fix for Mega code commit.
TFT LCD display
DiveIno uses the 3.2 inch TFT IPS 480 x 320 262K Color Full-Angle LCD Module. It is basically a shield, which can be mounted on top of the Arduino Mega board. The pins, which are used by this module is on the same place on Mega and Due, so the shield can be easily plugged onto the Arduino Due board.
It will work, because the UTFT library supports both modules.
SD card
The SD card slot is mounted on the back of the TFT LCD shield. It uses SPI protocol for communication with the microcontroller board. The pins which supports it are the followings:
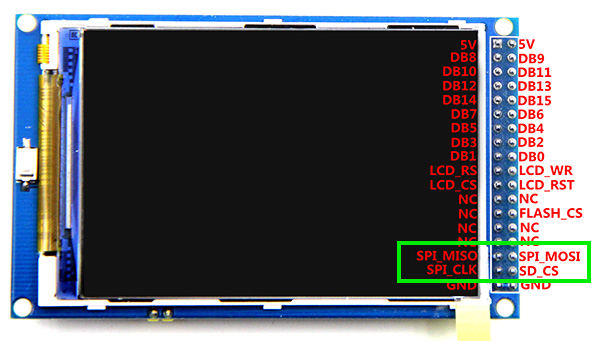
This will work fine for Arduino Mega, but Arduino Due has an SPI header in the middle of the board:
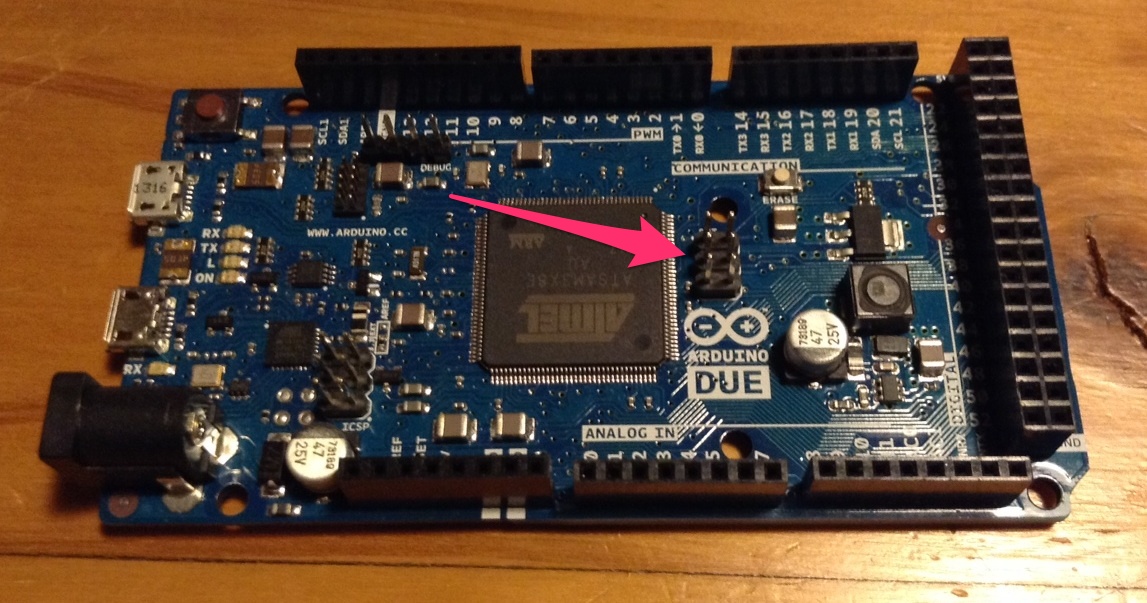
It means that the TFT LCD shield mounted SD card slot doesn’t work out of the box for Arduino Due. The following solutions are possible:
- Modify the TFT LCD shield to connect into the on board Due SPI header
- Use a separate Micro SD card module
For the time being I picked the second option. The Micro SD Card Storage Memory Board Module was used instead the TFT LCD mounted one. This is the reason why a different CS pin was used for Mega and Due:
1 |
|
Final layout
As a migration result the Arduino Due based DiveIno version looks like this on a solderless breadboard:
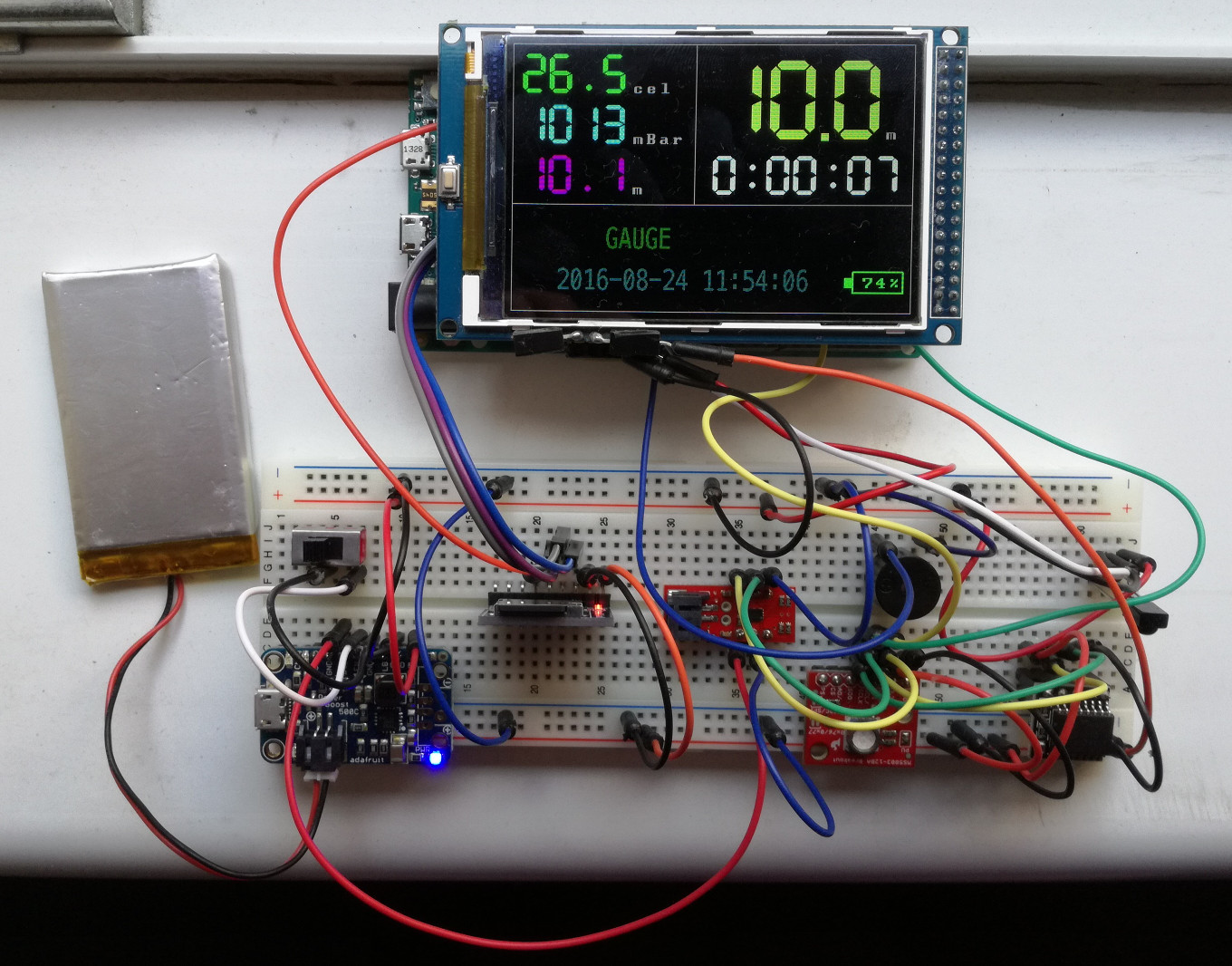
All parts should look familiar to you except the new Micro SD card module in the middle.